
Mastering Git: 5 Tips for Efficient Version Control
What’s Git?
First CTA: Download git. It’s a widely used distributed version control system for tracking changes in source code. A difference between the other VCS is the way data is processed.
It allows developing teams to collaborate, making it easier to manage and merge code. It was created by Linus Torvalds 🇫🇮 in 2005 and has since become a popular VCS.
It provides a decentralized approach, which means that each developer has their local copy of the entire repository, including its complete history.
It uses data like a series of snapshots of mini-filesystem. Every time you commit, or save the state of your project, it takes a picture of your files and stores a reference to it.
Key features include
- Committing: it tracks changes through commits, which are snapshots of the project’s state. Developers commit their changes along with a descriptive message [modifications made].
- Branching and Merging: it allows you to create branches to isolate changes and work on new features or bug fixes independently. Branches can later be merged.
- History and Time Travel: it preserves the complete history of a project, allowing you to navigate through commits, view and revert changes if necessary facilitating troubleshooting.
- Collaboration and Remote Repositories: it supports collaboration among teams by providing mechanisms for sharing changes and, to pull and push to git.
- Version Tagging: it allows for version tagging, enabling you to mark specific points in the project’s history as significant milestones.
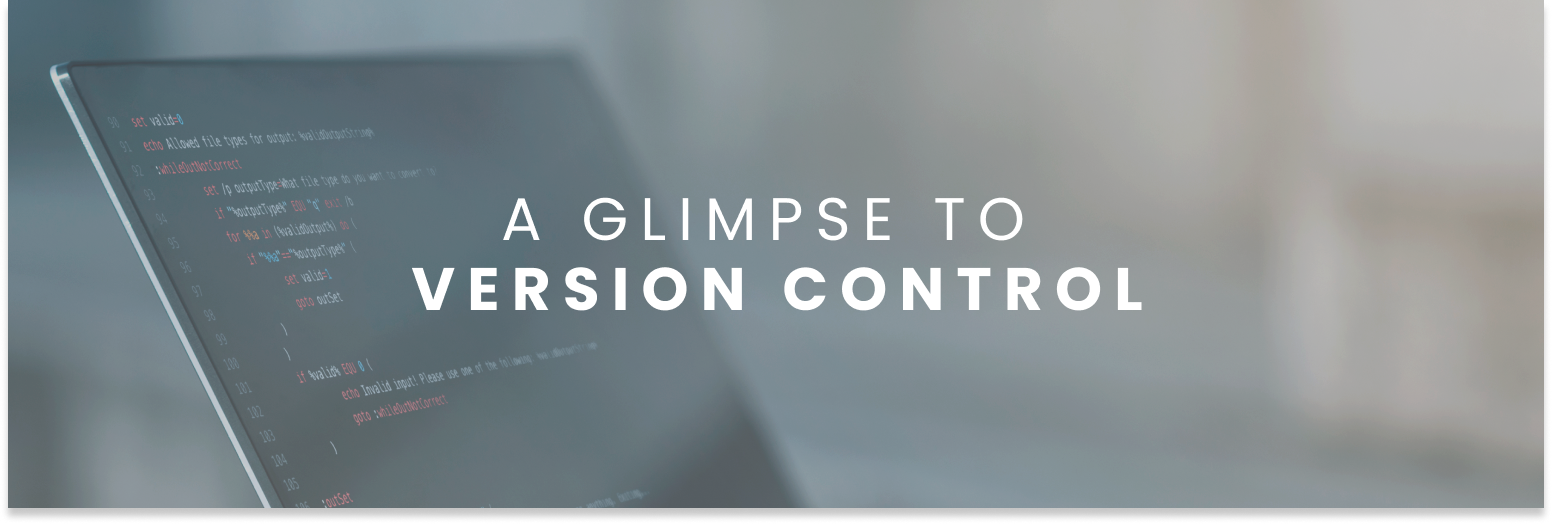
Checkout Git, it provides a wide range of benefits, including:
- Allowing easy rollback to previous versions if needed.
- Facilitating code review and providing a clear audit trail.
- Supporting experimentation and feature development in separate branches.
Tips you need to bear in mind:
- Commit Early and Frequently
- Make it a habit! Commit changes regularly. It allows you to capture incremental progress making it easier to revert changes or follow up on issues.
- Write Descriptive Commit Messages:
- A well-written message helps understand the change context and intention. Be succinct, use AI.
- Use Branches for New Features and Experiments:
- Git branching: use new features or experiments separately. Keeping main branch clean and stable while developing and testing new functionality.
- Regularly Pull and Push to Remote Repository:
- Check out git for changes made by pulling updates from the remote repository. Pull the latest changes to ensure you have the most up-to-date codebase.
- Learn Essential Commands:
git status
[to see the status of your repository]
git add
[to stage changes for a commit]
git commit
[to create a commit]
git pull
[to fetch and merge changes from a remote repository]
git push
[to push your local commits to a remote repository]
git stash
[to save changes that you have made without committing them]
Here’s a brief overview of how `git stash` works:
- When you run
git stash
, it saves your modified tracked files and staged changes into a new stash, leaving your working directory clean.
- You can give the stash a name or leave it unnamed.
- You can continue working on your branch, switch to another branch, or perform other Git operations.
- Later, when you’re ready to apply the changes saved in the stash, you can use
git stash apply
or git stash pop
-
git stash apply
applies the changes from the most recent stash while keeping the stash intact.
git stash pop
applies the changes from the most recent stash and removes it from the stash list.
- If you have multiple stashes, you can specify a stash by its index or name when using
git stash apply
or git stash pop
.
- You can also use
git stash list
to view the list of stashes you have created.
Git Basics
CVCS and Distributed Version Control Systems [DVCS] differ in their architecture and how they handle collaboration and version control.
Why has it become the de facto standard?
- Performance and Scalability: designed to be fast, even with large repositories and extensive history. It employs various techniques, such as efficient data storage and diff algorithms, to optimize performance.
- Distributed Nature: distributed architecture enables branching and merging, facilitating workflows.
- Strong Community and Ecosystem: a large and active community, contributing, providing support, and creating a vast ecosystem of tools, integrations, and hosting platforms.
- Popularity and Adoption: with many high-profile projects and organizations adopting it; has led to a network effect, making it the de facto standard for VC.
- GitHub: it played a significant role in its rise to prominence. Its user-friendly interface, collaboration features, and integrations made it more accessible and appealing to developers and teams.
These factors, along with it’s flexibility have contributed to its dominance as the preferred version control system for many developers and organizations.
Setting Up Git
- Install Git:
- Download the appropriate installer for your operating system from the official website.
- Run the installer and follow the on-screen instructions.
- During the installation, you can choose the components to install and the default settings. The default options are usually sufficient for most users.
- Configure it:
- Open a terminal or command prompt.
- Set your username by running the following command, replacing “Your Name” with your actual name:
git config --global user.name "Your Name"
Set your email address by running the following command:
git config --global user.email your-email@example.com
You can also configure other settings such as: preferred text editor and default branch name.
Verify the Installation:
To verify that installing Git is correctly done, run the following command in the terminal:
If Git installation is properly done, it will display the installed version of it.
- Set Up SSH Keys [Recommended]:
- Generating SSH keys allows you to securely authenticate with remote repositories without entering your username and password.
- Follow the instructions in the official GitHub guide to generate and add your SSH key.
Once you have completed these steps, get ready to fly!
To keep in mind: If you prefer a graphical user interface (GUI) for it, there are several available, such as GitHub Desktop, Sourcetree, and GitKraken.

- Creating Repositories:
- They can be created locally on your machine or hosted on a remote server. Use a hosting platform like Hub, Lab, or Bitbucket.
- To create a local repository, navigate to the directory where you want to create the repository and use the command
git init
. This initializes a new repository in the current directory.
- Initializing a New Repository:
- To initialize a new repository locally, navigate to the project’s directory in your command-line interface and run the command
git init
.
- This creates a hidden
.git
directory that contains all the necessary metadata and tracking information for version control.
- Cloning an Existing Repository:
- Cloning allows you to create a local copy of an existing repository.
- To clone a repository, use the command
git clone <repository_url>
, where <repository_url>
is the URL of the repository you want to clone.
- Will create a new directory with the same name as the repository and copy all the files and commit history into it.
- Creating Branches:
- Branches allow for independent lines of development. You can create a new branch to work on a specific feature or bug fix without affecting the main branch.
- To create a new branch, use the command
git branch <branch_name>
. For example, git branch feature-branch
creates a new branch named “feature-branch”.
- Mostly used:
git checkout -b <branch_name>
Faster and straightforward way to take you to the new branch.
$ git checkout -b feature-branch
Switched to a new branch 'feature-branch'
- Switching Between Branches:
- To switch to a different branch, use the command
git checkout <branch_name>
. For example, git checkout feature-branch
switches to the “feature-branch” branch.
- After switching branches, your working directory will reflect the state of the branch you switched to.
- Merging Branches:
- To incorporate changes from one branch into another, you can merge branches.
- First, ensure you are on the branch where you want to merge the changes. For example, to merge the changes from “feature-branch” into the current branch, use
git checkout main
.
- Then, run the command
git merge <branch_name>
, where <branch_name>
is the name of the branch you want to merge into the current branch. For example, git merge feature-branch
.
- It will attempt to automatically merge the changes. If conflicts occur, you’ll need to manually resolve them.
- After successful merging, the changes from the other branch are incorporated into the current branch.
Its workflow outlined above covers the basic steps involved in creating repositories, initializing a new repository, cloning an existing repository, creating branches, switching between branches, and merging branches.
These are fundamental concepts that allow for effective collaboration and version control.
Working with Commits
- Creating Commits:
- After making changes to your project files, you can create a commit to record those changes.
- First, add the changes to the staging area using
git add <file_name>
or git add .
to add all modified files.
- To create a commit, use the command
git commit -m "commit message"
. Replace “commit message” with a descriptive message that explains the changes made in the commit.
- The commit is now recorded in the repository, capturing a snapshot of the project at that point in time.
- Viewing Commit History:
- To view the commit history of a repository, use the command
git log
.
- The
git log
command displays a list of commits, including the commit hash, author, date, and commit message.
- By default,
git log
shows the commits in reverse chronological order.
- You can use various options and filters with
git log
to customize the output, such as limiting the number of commits displayed or filtering by author or date.
- Making Changes to Existing Commits:
- Commits are intended to be immutable and represent a specific snapshot of the project. However, there are ways to make changes to existing commits.
- If you need to modify the most recent commit (e.g., correcting a typo in the commit message or adding a missed file), you can use the command
git commit --amend
.
- This command allows you to modify the previous commit by amending the changes or updating the commit message.
- If you need to make changes to an older commit, you can use interactive rebase or other advanced techniques. These methods involve rewriting the commit history and should be used with caution, especially if the commits have already been shared with others.
- Discarding Local Changes:
- If you have made changes to your project but decide not to commit them, you can discard those changes and revert back to the last committed state.
- Use
git checkout -- <file_name>
to discard changes in a specific file, or git checkout .
to discard changes in all files.
- Be cautious when using
git checkout --
as it permanently discards the changes.
Working with commits in allows you to track the history of your project, review changes, and collaborate effectively with other developers.
It’s important to create descriptive commit messages that accurately describe the changes made in each commit, making it easier to understand and navigate the project’s history.
To eliminate changes made locally, even the ones in stagging [previous to the commit process], you can use git reset hard. Meaning, if you have uncommitted changes, but you did an add, will be eliminated.
In other words git reset –hard, AKA as a hard reset, updates the current branch tip to the specified commit, unstages any changes, and also deletes any changes from the working directory.
Branching and Merging
Dive into the power of branching and merging. Explore the benefits of creating branches for different features or bug fixes, and how to merge branches back into the main branch. Follow this link.
Remote Repositories
Visual aid! The concept of remote repositories helps demonstrate how to work with remote repositories using commands like git clone
, git push
, and git pull
.
Find how to collaborate with others using remote repositories and how to handle common remote-related tasks.
Git Best Practices
If you feel like sharing best practices you’ve had when using it, please be our guest!

Git Bash is a command-line interface (CLI) program that provides an emulation layer for running its commands on a Windows operating system.
It combines the functionality of it, which is a distributed version control system, with the Unix-like command line environment provided by Bash (Bourne Again SHell).
It allows Windows users to access and use its commands in a familiar Unix-like environment, which is particularly useful for developers who are accustomed to working with systems like Linux or macOS.
It provides a consistent command-line experience across different platforms, enabling Windows users to work seamlessly with its repositories.
It also supports standard Bash commands and provides a set of Unix utilities commonly used in command-line workflows. This includes file and directory manipulation, text processing tools, and scripting capabilities.
This makes it a versatile tool for developers and power users who prefer to work with the command line for version control and other tasks.
It can be installed as part of the Git for Windows package, which bundles it along with a Bash emulation layer.
Once installed, users can launch it to access the command-line interface and execute commands, navigate directories, run scripts, and perform various tasks using a combination with Bash functionality.
A Quick Round Up
Want to be part of our community? Work with us!
0 thoughts on “Mastering Git: 5 Tips for Efficient Version Control”
Leave a Reply
You must be logged in to post a comment.